Create a non-Genero client for a JSON Web service
In order to consume a BAM-generated JSON Web service, a developer must understand the format of the HTTP REST JSON operations (requests and responses).
Before you begin, get the WebService_serviceclient.4gl file from the Web Service provider. Although written in Genero BDL, this file contains the information needed to create the JSON body for the requests and responses. Consider this file as the documentation of how the Web service works.
- The URL of the REST resource.
- The HTTP header, to include the HTTP method, additional REST resource, and a possible CRUD
operation.
- The HTTP method will be either
GET
,PUT
,POST
, orDELETE
. - The resource provides additional URL details that specify which record is involved in the request
- Some CRUD operations require you to provide the
CRUDOperation
in the header, specifically when you read, update or delete a collection.
- The HTTP method will be either
- The request body and response body. Data is exchanged in JSON format over HTTP. Examine the
WebService_serviceclient.4gl
file to identify what is expected for both the request AND the response.
- The request body provides the data that the Web service needs to do its
work. In the
WebService_serviceclient.4gl
file, this is managed using a record with the suffix
_IN_type
. - The response body provides the client application with the status of the
operation (where 200 indicates success) along with any additional data
sent back by the service. In the
WebService_serviceclient.4gl
file, this is managed using a record with the suffix
_OUT_type
.
- The request body provides the data that the Web service needs to do its
work. In the
WebService_serviceclient.4gl
file, this is managed using a record with the suffix
URL of the REST resource
The URL of the REST resource takes the following format:
http://<server>/<alias>/ws/r/<group>/REST/<resource>[(<IDs>)]
<server>
- Service server name or IP address.<alias>
- fastcgi / http dispatcher configuration<group>
- Web service server, for example, GAS group configuration or external configuration filename<resource>
- Web service entity- <IDs> (optional) - List of identifiers for records. IDs are enclosed by single quotes and separated by commas.
JSON Service example
The Customers web service example used for these topics can be found under the Four Js Genero github repository. See http://github.com/FourjsGenero/ex_bam_json_web_service
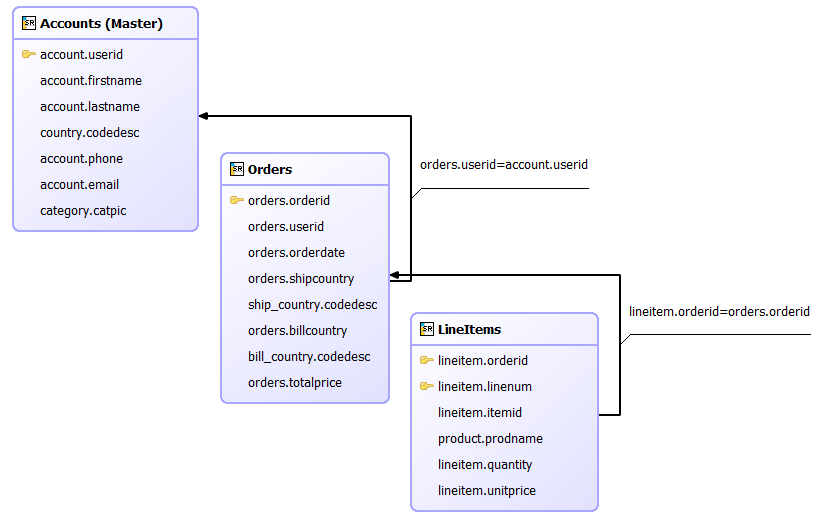
Functional description | URL of the REST resource | Scope | HTTP Method | CRUD operation | Request Body | Response Body |
---|---|---|---|---|---|---|
Returns a list of all customers (i.e., accounts + orders + lineitems) |
http://localhost:8090/ws/r/JSONServer/
|
Collection | POST | READCollection | Query By Example | Error number + Collections of customers |
Return the account "dupont" | http://localhost:8090/ws/r/JSONServer/
|
Item | GET | Primary Key | Error number + Account data for customer dupont | |
Return the order 1 | http://localhost:8090/ws/r/JSONServer/
|
Item | GET | Primary Key | Error number + Order data for order 1 | |
Delete a list of accounts | http://localhost:8090/ws/r/JSONServer/
|
Collection | POST | DELETECollection | Array of Keys | Error number |
Delete the account "dupont" | http://localhost:8090/ws/r/JSONServer/
|
Item | DELETE | Primary Key | Error number | |
Update a list of accounts | http://localhost:8090/ws/r/JSONServer/
|
Collection | PUT | UPDATECollection | Array of Data | Error number |
Update the account "dupont" | http://localhost:8090/ws/r/JSONServer/
|
Item | PUT | Data | Error number | |
Create a document (createAll) | http://localhost:8090/ws/r/JSONServer/
|
Collection | POST | Array of Data | Error number + collection of keys | |
Create a list of accounts | http://localhost:8090/ws/r/JSONServer/
|
Collection | POST | Array of Data | Error number + collection of keys |
JSON resources
The implementation of a JSON Item of the Business Application diagram defines a data set. In a data set, each record defines one resource and provides methods to create, read, update, and delete data.
Table 2 shows the resources defined for the JSON item Customers of our example. This item provides three records, Accounts, Orders, and LineItems.
Resource | URI |
---|---|
Customers | /Customers |
Accounts | /Customers/Accounts |
Orders | /Customers/Accounts/Orders |
LineItems | /Customers/Accounts/Orders/LineItems |
HTTP header | ||||
---|---|---|---|---|
Description | Related function | Request record | Response record | |
POST /Customers |
||||
Creates a collection of documents. | Customers_client_createAll() |
createAll_IN_type |
createAll_OUT_type |
|
POST
/Customers
|
||||
Reads a collection of documents from a Query by Example (QBE). | Customers_client_readAll() |
readAll_IN_type |
readAll_OUT_type |
|
POST /Customers/Accounts |
||||
Creates a collection of new accounts. | Customers_client_Accounts_create() |
Accounts_create_IN_type |
Accounts_create_OUT_type |
|
GET
/Customers/Accounts('accountID') |
||||
Reads the account specified by accountID. | Customers_client_Accounts_readRow() |
Accounts_ws_br_uk_type |
Accounts_read_OUT_type |
|
PUT
/Customers/Accounts
|
||||
Updates a collection of accounts | Customers_client_Accounts_update() |
Accounts_update_IN_type |
Accounts_update_OUT_type |
|
PUT
/Customers/Accounts('accountID') |
||||
Updates the account specified by accountID. | Customers_client_Accounts_updateRow() |
Accounts_ws_br_uk_type |
Accounts_update_OUT_type |
|
POST
/Customers/Accounts
|
||||
Deletes a collection of accounts | Customers_client_Accounts_delete() |
Accounts_delete_IN_type |
Accounts_delete_OUT_type |
|
DELETE
/Customers/Accounts('accountID') |
||||
Deletes the account specified by accountID. | Customers_client_Accounts_deleteRow() |
Accounts_ws_br_uk_type |
Accounts_delete_OUT_type |
|
POST /Customers/Accounts/Orders |
||||
Creates a collection of new orders. | Customers_client__Orders_create() |
Orders_create_IN_type |
Orders_create_OUT_type |
|
GET
/Customers/Accounts/Orders('orderID') |
||||
Reads the order specified by orderID. | Customers_client__Orders_readRow() |
Orders_ws_br_uk_type |
Orders_read_OUT_type |
|
PUT
/Customers/Accounts/Orders
|
||||
Updates a collection of orders | Customers_client__Orders_update() |
Orders_update_IN_type |
Orders_update_OUT_type |
|
PUT
/Customers/Accounts/Orders('orderID') |
||||
Updates the order specified by orderID. | Customers_client__Orders_updateRow() |
Orders_ws_br_uk_type |
Orders_update_OUT_type |
|
POST
/Customers/Accounts/Orders
|
||||
Deletes a collection of orders | Customers_client__Orders_delete() |
Orders_delete_IN_type |
Orders_delete_OUT_type |
|
DELETE
/Customers/Accounts/Orders/LineItems('lineItemID') |
||||
Deletes the line item specified by lineItemID. | Customers_client__Orders_deleteRow() |
Orders_ws_br_uk_type |
Orders_delete_OUT_type |
|
POST
/Customers/Accounts/Orders/LineItems |
||||
Creates a collection of new line items. | Customers_client__LineItems_create() |
LineItems_create_IN_type |
LineItems_create_OUT_type |
|
GET
/Customers/Accounts/Orders/LineItems('lineItemID') |
||||
Reads the line item specified by lineItemID. | Customers_client__LineItems_readRow() |
LineItems_ws_br_uk_type |
LineItems_read_OUT_type |
|
PUT
/Customers/Accounts/Orders/LineItems
|
||||
Updates a collection of line items | Customers_client__LineItems_update() |
LineItems_update_IN_type |
LineItems_update_OUT_type |
|
PUT
/Customers/Accounts/Orders/LineItems('lineItemID') |
||||
Updates the line item specified by lineItemID. | Customers_client__LineItems_updateRow() |
LineItems_ws_br_uk_type |
LineItems_update_OUT_type |
|
POST
/Customers/Accounts/Orders/LineItems
|
||||
Deletes a collection of line items | Customers_client__LineItems_delete() |
Orders_delete_IN_type |
Orders_delete_OUT_type |
|
DELETE
/Customers/Accounts/Orders/LineItems(''lineItemID') |
||||
Deletes the line item specified by lineItemID. | Customers_client__LineItems_deleteRow() |
Orders_ws_br_uk_type |
Orders_delete_OUT_type |