Download a file as an attachment to a response
This example demonstrates how to return a file as an attachment using the
WSAttachment
attribute
In order to return a file as an attachment to a client, set the WSAttachment attribute on an output parameter.
Example: downloading file using WSAttachment
- A return paramater is defined as
STRING
type with aWSAttachment
attribute. The REST engine treats the parameter value as a path to a file to be attached. - A
WSMedia
attribute is added to handle the data format for images. The wildcard (image/*
) in WSMedia allows for all image types. If the file can be any type,WSMedia
is not specified withWSAttachment
.
The format is chosen according to that specified in
the Accept
or Content-Type
headers. You code in your
function to replace the image/*
placeholder in the Accept
or Content-Type
header with the actual value. This can also be done using
the WSContext attribute to set the header.
WSError
attributed variable is set
with a description of the error and the SetRestError()
method
is called to return it.IMPORT com
IMPORT os
PUBLIC DEFINE userError RECORD ATTRIBUTES(WSError = "User error")
message STRING
END RECORD
PUBLIC FUNCTION downloadImageFile()
ATTRIBUTES (WSGet,
WSPath = "/files3/images",
WSDescription = "download image file to the client with WSAttachment",
WSThrows = "400:@userError")
RETURNS (STRING ATTRIBUTES(WSAttachment, WSMedia = "image/*") )
DEFINE ret, fname STRING
DEFINE ok INTEGER
LET fname = "favicon.ico"
LET ok = os.Path.exists(fname)
IF ok THEN
LET ret = fname
ELSE
LET userError.message = SFMT("File (%1) does not exist", fname)
CALL com.WebServiceEngine.SetRestError(400,userError)
END IF
RETURN ret
END FUNCTION
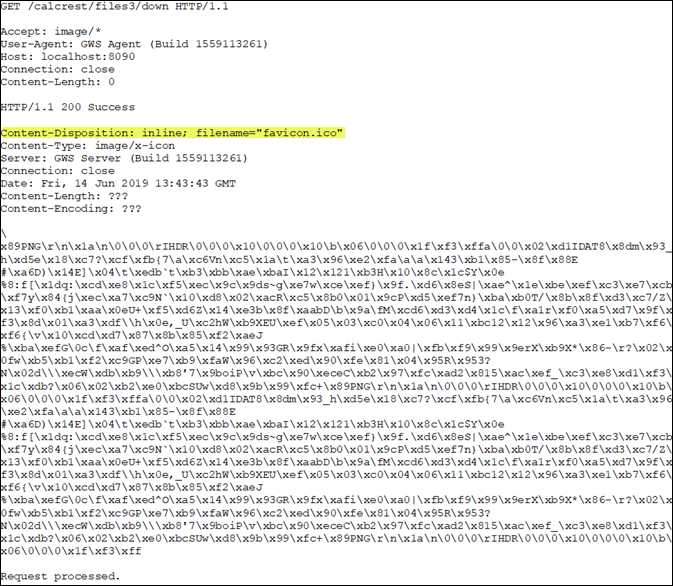
In Figure 1 the Content-Disposition
response header in the output
indicates that the content is expected as an attachment. Therefore, when the function is
called, the file is downloaded and saved to the client locally in its TMP
directory.