Example: upload a file in a multipart request
Shows an example of a method you might use from a client to upload an image along with other data in a function using a form-data type HTTP multipart request.
Example multipart request
- "id" is defined as type
INTEGER
. - "img" is defined as type
STRING
with aWSAttachment
attribute. The REST engine treats the parameter value as a path to a file to be attached. TheWSMedia
attribute handles the data format for images. - An input parameter "submit" is defined as type
STRING
.
The GWS stores the file in a temporary directory on the server, and returns the absolute path to the file in the input parameter ("img"). When the call to the function ends, the file will be removed unless it is saved to a location on disk.
The new_fname variable is set to create a path to the current directory, to which the filename is added with the os.Path.baseName instruction. The os.Path.rename instruction moves the file from the temporary directory to the current directory.
WSThrows – is set to handle
errors. If the id
is missing or there is a problem renaming and
moving the file, the message
field of the
userError
variable is set, and a call to SetRestError()
returns the message defined in
WSThrows
for the error.
IMPORT os
IMPORT com
PUBLIC DEFINE userError RECORD ATTRIBUTE(WSError = "User error")
message STRING
END RECORD
PUBLIC FUNCTION fetchFiles(
id INTEGER,
img STRING ATTRIBUTE(WSAttachment,WSMedia = "image/*"),
submit STRING
)
ATTRIBUTES (WSPost,
WSPath = "/files/fetch",
WSDescription = "upload image file to the server from browser",
WSThrows = "400:@userError")
RETURNS STRING ATTRIBUTE(WSMedia = "text/html")
DEFINE ret, new_fname STRING
DEFINE ok INTEGER
IF id == 0 THEN
LET userError.message = "Missing id"
LET ret = SFMT("<HTML><body><h1>Must have an ID %1</h1></body></HTML>",id)
CALL com.WebServiceEngine.SetRestError(400,userError)
ELSE
TRY
LET new_fname = os.Path.baseName(img)
LET new_fname = os.Path.pwd()||"/"||new_fname
LET ok = os.Path.rename(img,new_fname)
LET ret = SFMT("Got image with ID %1",id)
CATCH
LET userError.message = "Error uploading file"
LET ret = SFMT("Error uploading file %1",id)
CALL com.WebServiceEngine.SetRestError(400,userError)
END TRY
END IF
RETURN ret
END FUNCTION
Create a client side HTML page to upload file
- In a text editor, create the form from the code sample. Remember to change
the
action
tag value to the URL of your server. - Save the file to a .html file, for example, upload.html, and place it on the client side.
- Open the file locally in a browser and select a file to upload. Ensure the service is running.
<!DOCTYPE html>
<HTML>
<form name = "Upload" method = "post"
action = "http://localhost:8090/Myservice/files/fetch"
enctype = "multipart/form-data">
<div>
<label for = "name">ID:</label>
<input type = "text" name = "id"/>
</div>
<div>
<label>Picture:</label>
<input type = "file" name = "img" accept = "image/png, image/jpeg" />
</div>
<input type = "submit" name = "submit" value="Send"/>
</form>
</HTML>
In the output the image is sent in parts. Parts are separated by boundaries, the
---------------------------70482736912266
On successfully receiving the file, the server returns the message to display in the browser. Otherwise, an error is returned and displayed.
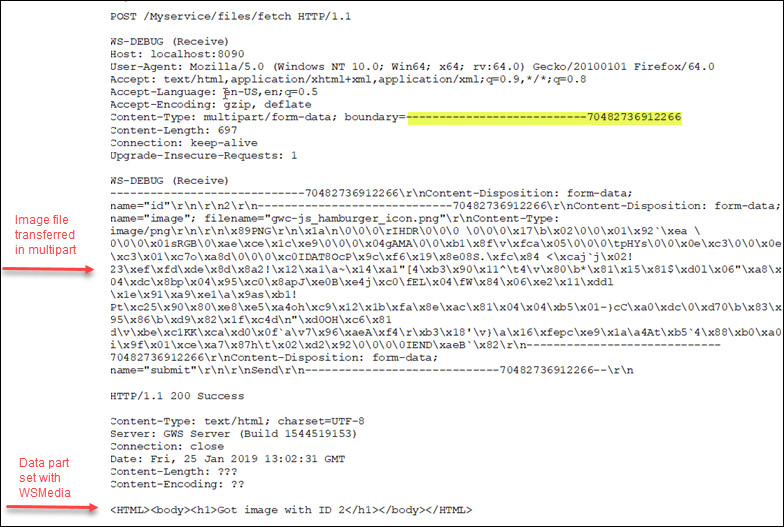