Example: upload a file in a multipart request
Shows an example of a method you might use from a client to upload an image along with other data in a function using a form-data type HTTP multipart request.
Example multipart request
The image file format is specified via the WSMedia
attribute. The attachment is
handled through the STRING
data type and the WSAttachment
and
WSMedia
attributes.
IMPORT os
IMPORT com
PUBLIC DEFINE myerror RECORD ATTRIBUTE(WSError="My error")
code INTEGER,
reason STRING
END RECORD
PUBLIC FUNCTION FetchFiles(
id INTEGER,
image STRING ATTRIBUTE(WSAttachment,WSMedia="image/*"),
submit STRING)
ATTRIBUTES (WSPost,
WSPath="/files/fetch",
WSDescription="upload image file to the server from browser",
WSThrows="400:@myerror")
RETURNS STRING ATTRIBUTE(WSMedia="text/html")
DEFINE ret, new_fname STRING
DEFINE ok INTEGER
IF id == 0 THEN
LET myerror.code=999
LET myerror.reason="Missing id"
LET ret = SFMT("<HTML><body><h1>Must have an ID %1</h1></body></HTML>",id)
CALL com.WebServiceEngine.SetRestError(400,myerror)
ELSE
TRY
LET new_fname = os.Path.baseName(image)
LET new_fname = os.Path.pwd()||"/"||new_fname
LET ok = os.Path.RENAME(image,new_fname)
LET ret = SFMT("Got image with ID %1",id)
CATCH
LET myerror.reason="Error uploading file"
LET ret = SFMT("Error uploading file %1",id)
CALL com.WebServiceEngine.SetRestError(400,myerror)
END TRY
END IF
RETURN ret
END FUNCTION
Create a client side HTML page to upload file
The "FetchFiles" function needs to be called by the client to upload a file. This example shows
you how to create a simple HTML page to select the file to upload. The HTML page contains a form
with two input fields and one submit button, according to the function's input
parameters.
- In a text editor, create the form from the code sample. Remember to change the
action
tag value to the URL of your server. - Save as, for example, upload.html on the client side.
- Open the file locally in a browser and select a file to upload. Ensure the service is running.
!DOCTYPE html>
<html>
<FORM NAME="upload" method="post" action="http://localhost:8090/Myservice/files/fetch" enctype='multipart/form-data'>
<div>
<label for="name">ID:</label>
<input type="text" name="id"/>
</div>
<div>
<label>picture:</label>
<input type="file" name="image" accept="image/png, image/jpeg" />
</div>
<input type="submit" name="submit" value="Send"/>
</FORM>
</html>
In the output the image is sent in parts. Parts are separated by boundaries, the
---------------------------70482736912266
On successfully receiving the file, the server returns the message to display in the browser. Otherwise, an error is returned and displayed.
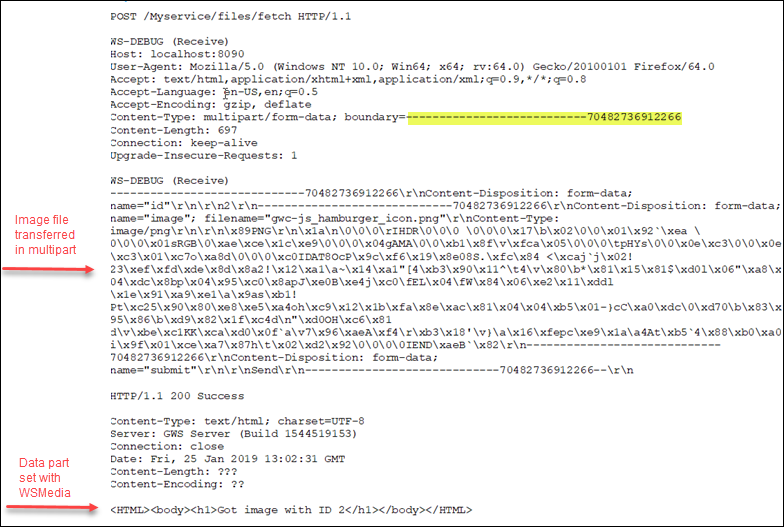